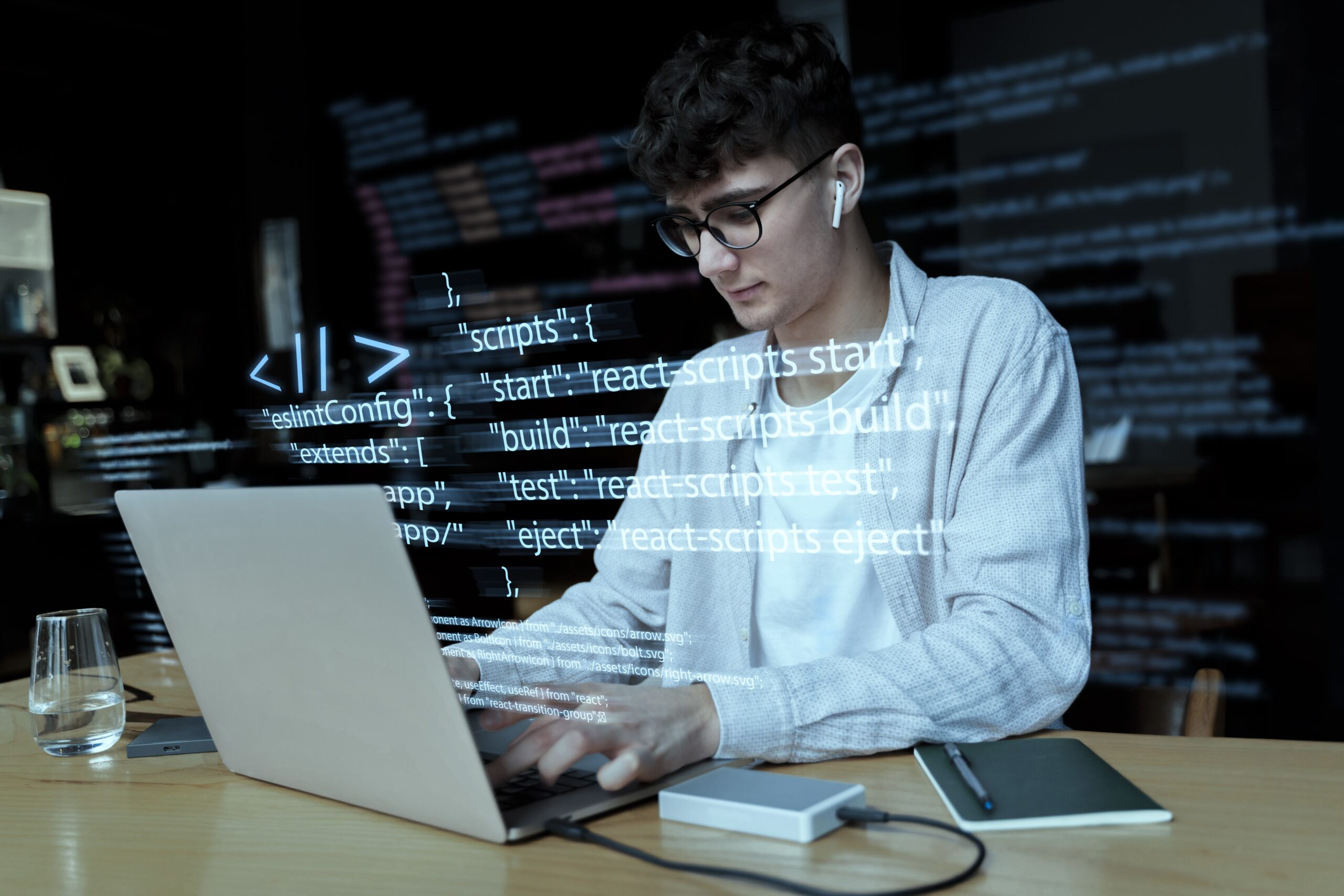
- Duration: 6 weeks
Categories: Programming
This Java course is designed to cover the essential concepts and features of Java programming, starting from basic syntax to advanced topics like multithreading, exception handling, and Java collections. You will gain a strong foundation in Object-Oriented Programming (OOP) principles and learn to build robust and scalable applications with real-world use cases.
Course Content Breakdown
Week 1: Java Basics and Object-Oriented Programming (OOP)
- Introduction to Java
- Overview of Java and its features.
- Setting up Java development environment (JDK, IDE).
- Understanding the Java syntax: Variables, data types, operators, and control flow (if-else, loops).
- Object-Oriented Programming (OOP)
- Classes and objects: Creating classes, constructors, and objects.
- Encapsulation: Using private variables and getters/setters.
- Inheritance: Extending classes, method overriding.
- Polymorphism: Method overloading and overriding.
- Abstraction: Abstract classes and interfaces.
- Hands-On Exercise
- Create a simple class for a bank account with methods to deposit and withdraw.
Week 2: Exception Handling and Strings
- Exception Handling in Java
- What are exceptions? Types of exceptions (checked vs. unchecked).
- Try-catch block and handling multiple exceptions.
- Throwing and catching custom exceptions.
- Using
finally
block for resource cleanup.
- Java Strings
- String class and its methods: Concatenation, comparison, and substring.
- StringBuffer and StringBuilder: Mutability and performance differences.
- Regular Expressions with String class.
- Hands-On Exercise
- Build a small program to validate user input and handle possible exceptions (e.g., invalid input, division by zero).
Week 3: Collections Framework
- Introduction to Java Collections
- What are collections? Why use collections in Java?
- The difference between arrays and collections.
- List Interface
- ArrayList, LinkedList: Insertion, deletion, and accessing elements.
- Iterating through a List using loops and iterators.
- Set Interface
- HashSet, LinkedHashSet, TreeSet: Unique elements and ordering.
- Map Interface
- HashMap, LinkedHashMap, TreeMap: Storing key-value pairs.
- Iterating over maps using entry set and key set.
- Hands-On Exercise
- Create a program to manage a collection of books using different collection types (e.g., List for storing books, Map for storing books with authors).
Week 4: Threads and Input/Output (I/O)
- Java Threads
- Introduction to multithreading and its importance.
- Creating threads using the
Thread
class andRunnable
interface. - Thread lifecycle and state transitions.
- Synchronization: Ensuring thread safety in shared resources.
- Deadlock prevention techniques.
- Input/Output in Java
- Working with InputStream and OutputStream.
- Reading and writing text files with FileReader and FileWriter.
- BufferedReader and BufferedWriter for efficient I/O operations.
- Serialization and Deserialization in Java (object persistence).
- Hands-On Exercise
- Implement a multithreaded program to download files concurrently.
- Write and read data from files using Java I/O classes.
Week 5: Advanced Concepts and Final Project
- Advanced Collection Features
- Using Generics in Collections for type safety.
- Concurrent collections: CopyOnWriteArrayList, ConcurrentHashMap.
- Sorting collections using Comparator and Comparable interfaces.
- Final Project
- Combine all learned concepts to develop a console-based student management system with:
- Handling student data using Collections.
- Exception handling for invalid inputs.
- Multi-threading to handle concurrent tasks like file reading and writing.
- I/O operations for data persistence.
- Combine all learned concepts to develop a console-based student management system with:
Why Choose TopCodder for Java Programming?
- Comprehensive Curriculum: Covers all essential Java concepts for real-world applications.
- Hands-On Learning: Practical exercises and real-world projects.
- Expert Trainers: Learn from professionals with industry experience.
- Certification: Get certified on completing the course to enhance your career prospects.
Leave feedback about this